useListener API
The useListener
hook enables you to perform actions in response to an event without stopping the event's propagation. This hook is useful when you want child component events to continue propagating to the application, while also updating the state of the parent component.
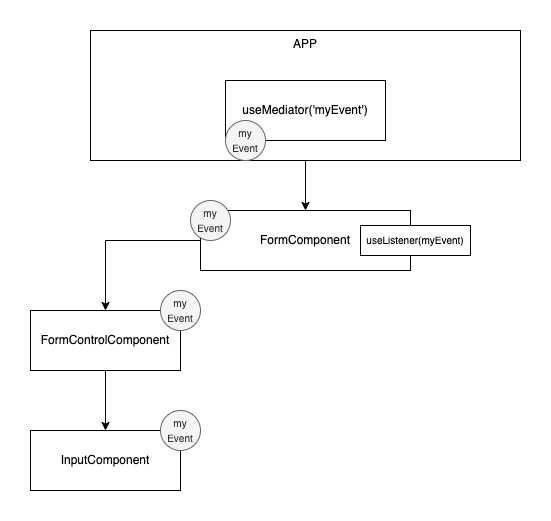
The useListener
hook functions similarly to useMediator
but allows event propagation. It is intended for use at the component level to manage internal state changes, such as UI updates. Do not use useListener
for handling events at the application level; in those cases, use useMediator
.
Parameters
The useListener
hook accepts three arguments:
useListener(eventName, eventHandler, HTMLElement);
-
eventName: The name of the event to listen for.
-
eventHandler: A function that processes the event payload. For example:
function handleMyEvent(payload) { console.log(payload); }
-
HTMLElement: The HTML element to which the event listener is attached.
Parameter | Description |
---|---|
eventName | Name of the event to listen for. |
eventHandler | Function to handle the event, accepting the payload as an argument. |
HTMLElement | HTML element where the listener will be attached. |
Example Usage
function ReactComponent() {
const ref = useRef();
function handleMyEvent() {
// React to the event here
}
useListener('myEvent', handleMyEvent, ref);
return <div ref={ref}>...</div>;
}